The Turtle module in Python allows you to draw with code. Woot!
Of course you’ll need to have Python installed on your local machine.
You’ll likely want to work with a code editor, of course, most of which support Python graphics. I use VSCode.
Step 1: Create a file with the extension .py on the end. Open it in your editor. Okay, that is two steps. (>.>).
Now you’ll want to make sure you include the turtle module with
and close that out with import turtle
at the end. turtle.done()
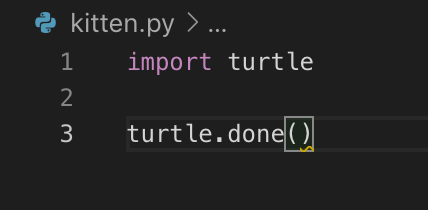
Next, right under
make a new instance of a turtle like so (the second turtle uses a capital T): import turtle
kitten = turtle.Turtle()
Then you can go ahead and tell it to move!
Draw a line forward 100 pixels:
kitten.forward(100)
Turn left 45 degrees:
kitten.left(45)
Turn right 90 degrees:
kitten.right(90)
Seems pretty logical, right?
Here is how we can make a square:
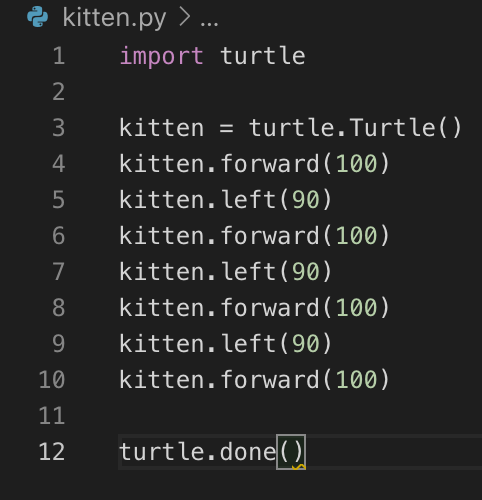
Make the turtle and line a color:
kitten.color("cyan")
Or you could even use an RGB or hex value:
kitten.color("#ffffff")
If you make it cyan it will look like this at the end of the animation:
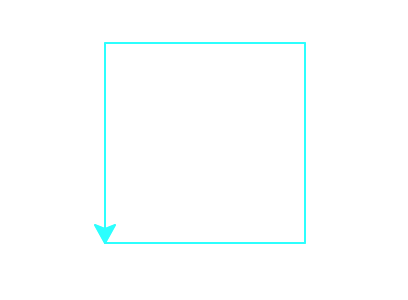
If you wrap your code with a
and kitten.begin_fill()
, the square will turn blue at the end of the animation. kitten.end_fill()
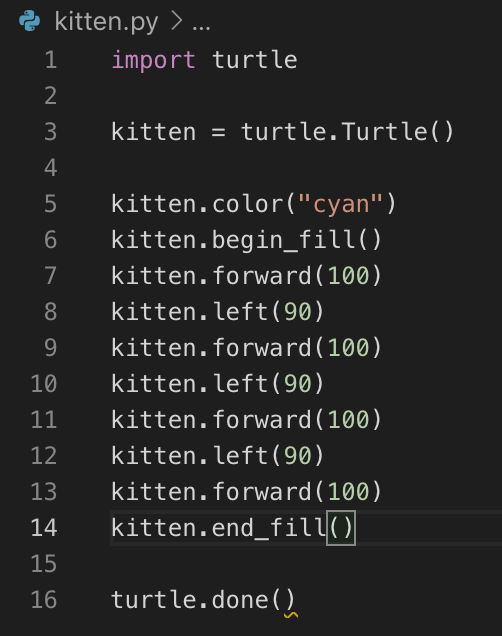
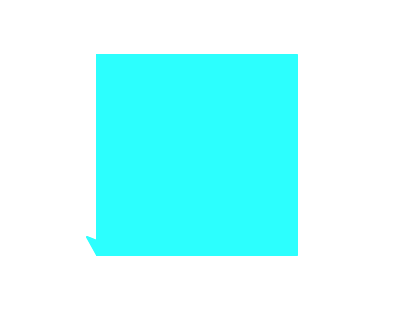
You can make the border cyan and the fill pink like so:
kitten.color("cyan", "pink")
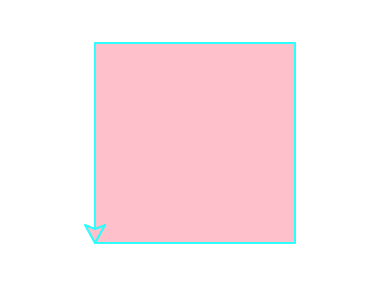
It’s cute! Hooray! We can draw a square! We just made it through our first day of Python Preschool. Woot!